· Ricardo Batista · 12 min read
Google Calendar API - How to sync your events easily
Google Calendar API guide with OAuth2 steps, syncing tricks, and quick fixes - simple examples and practical debug tips for real projects.
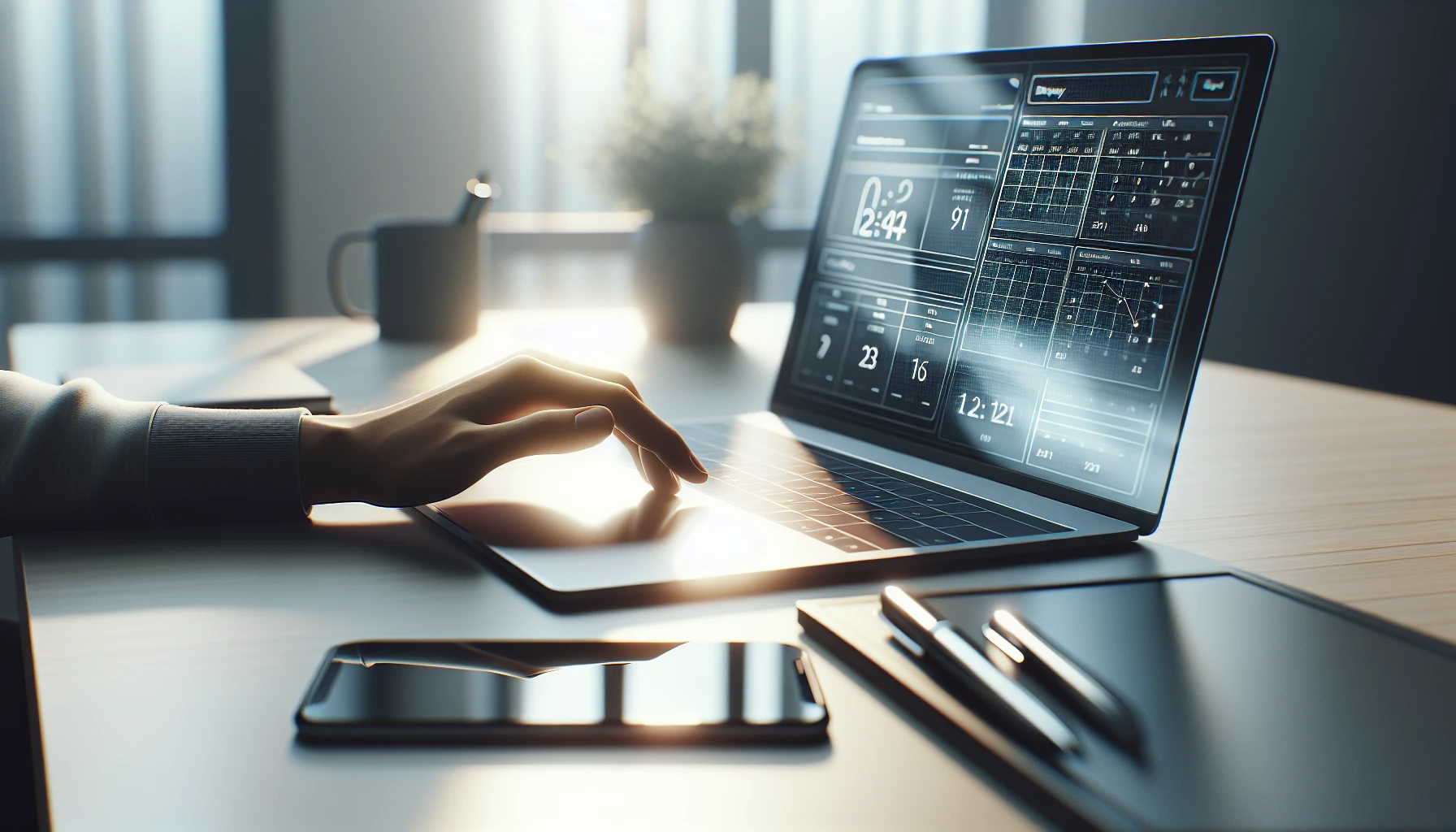
Discovering how to use Google Calendar API might be a bit messy at first, but it opens affordable access to dynamic scheduling and event tracking. The guide covers OAuth2 steps and Google Developer Console setups, with hints from Stack Overflow and Chrome Developer tools. It provides a mix of practical tips and clear directions, making things accessible and fun.
Key Takeaways
- The Google Calendar API is a handy tool for managing your events and calendars smoothly
- Setting up OAuth2 might seem tricky at first but following clear steps makes it easier
- We show our expertise as Meeting Reminders automatically ping the attendees that arrive late to your meetings, keeping things on track
- Troubleshooting common issues like wrong credentials or tokens is all part of getting the system running right
- Integrating the API with other tools and platforms can boost your productivity, making your workflow just a bit more streamlined
Google Calendar API Overview and Setup Using OAuth2 and Google Developer Console
The Google Calendar API gives you the power to create, update, and manage calendar events. It is great for meeting reminders, such as automatically pinging attendees who arrive late to meetings. The API makes it simple to integrate event reminders with your existing applications. Getting started with this API needs a few clear steps, using OAuth2 for secure access and the Google Developer Console for configuration.
Understanding OAuth2
OAuth2 is the protocol that allows your app to access user data for the Google Calendar API safely. Instead of saving user credentials inside your app, you use tokens, making the whole process safer. Here’s a brief explanation of how it works:
- The user connects their calendar to your app.
- Google validates the connection through OAuth2.
- Your application gets a token that can be used in API calls.
- The token expires after a certain period, so you must refresh it when needed.
Using OAuth2 means a lower risk of security issues compared with storing passwords or sensitive data directly. For detailed setup instructions, visit Google Developer Console.
Steps to Set Up the API
- Sign in to the Google Developer Console.
- Create a new project.
- Enable the Google Calendar API for your project.
- Configure the OAuth2 consent screen with the necessary scopes.
- Create client credentials.
- Download the client secret JSON file.
- Use the client ID and secret in your code to start the OAuth2 flow.
Following these steps is straightforward once you get the hang of the system. Many developers benefit from a mix of clear documentation and hands-on practice.
Troubleshooting Typical Errors and Glitches
When working with the Google Calendar API, you might run into errors. Issues like permission problems or token expiration can slow down development if not handled properly. Checking out resources like Stack Overflow can help with practical fixes and workarounds.
Common Problems and Fixes
- Invalid Credentials:
Make sure your OAuth2 tokens are correctly generated and have not expired. Double-check client IDs and secrets. - Permission Denied:
Confirm that the scopes provided in the OAuth2 consent screen are correct. Sometimes additional permissions are needed if you require access to calendar events that the user might not be familiar with. - API Rate Limits:
If you see errors about too many requests, consider implementing exponential backoff to avoid overwhelming the API. - Debugging Tips:
Use Chrome DevTools to inspect API calls. This tool helps to see network requests in real time, check payloads, and identify issues in the front-end code. Learn more about debugging with Chrome DevTools.
Practical Handling of Errors
When encountering an error:
- Verify that your OAuth2 settings in the Developer Console match what your application expects.
- Utilize the debug tools in Chrome DevTools to inspect the request and response headers.
- Search for similar issues on Stack Overflow; many developers have already encountered and fixed these problems.
Practical Integration Examples
Integrating the Google Calendar API with popular platforms can enhance the meeting reminder system in innovative ways. Below are a few integration examples using SpringBoot, a Bubble API connector, and the Zoom meetings API.
Integration with SpringBoot
SpringBoot is a popular framework for building Java applications. It provides an easy way to create web services, and its dependency injection makes the integration smooth.
Example Steps:
- Add the Google Calendar API dependency to your Maven or Gradle project.
- Configure OAuth2 settings in SpringBoot using Spring Security.
- Create an endpoint that listens for calendar event updates.
- When a meeting event is about to start, your application checks if attendees are late and sends reminder notifications.
This approach ensures that reminders are triggered precisely when needed, reducing the chances of missing meetings or forgetting updates.
Integration using Bubble API Connector
For those who prefer a no-code environment, Bubble offers an API connector that can integrate with the Google Calendar API. It is an attractive solution if you want to build workflows without in-depth programming knowledge.
Key Points:
- Configure the API URL and authentication parameters in Bubble.
- Set up conditions to trigger reminders before or after scheduled meetings.
- Use Bubble’s workflow engine to route the API responses and trigger appropriate actions like emails or in-app notifications.
This method is particularly beneficial for startups or small teams that need rapid development cycles without heavy coding.
Integration with Zoom Meetings API
Linking the Google Calendar API with the Zoom meetings API allows for bi-directional synchronization. This way, when a meeting is scheduled in Zoom, it also appears in your Google Calendar with built-in reminder features.
Implementation Outline:
- Authenticate both the Google Calendar and Zoom APIs using OAuth2.
- Set up webhook listeners in your application to capture Zoom meeting events.
- On receiving a meeting event, update the corresponding Google Calendar entry.
- If an attendee is late, the system can automatically send a reminder email or push notification.
This bi-directional sync ensures that meeting reminders remain up-to-date across platforms, reducing confusion and keeping all participants informed on any schedule changes.
Custom Add-on Creation with Google Apps Script
Google Apps Script offers a simple way to build custom add-ons that work directly with Google Calendar. These add-ons can enhance meeting reminder capabilities and provide custom actions right from Google’s interface.
Getting Started with Apps Script
Google Apps Script uses JavaScript-like syntax which makes it accessible even if you’re not a seasoned programmer.
Step-by-Step:
- Open the Google Apps Script editor from your Google Calendar.
- Write a script to add a custom menu. For example, you can add a “Send Late Reminder” option that iterates through upcoming events.
- Use the CalendarApp service to fetch events and check attendee status.
- Write functions that send notifications based on custom conditions (e.g., if an attendee statistically tends to be late).
Using Google Apps Script is ideal if you want quick prototyping or lightweight enhancements without setting up a full backend service.
- Advantages of custom add-ons include:
- Direct integration with the Google Calendar interface.
- No need for external hosting.
- Rapid updates and changes using built-in version control.
Client-side Techniques with Mustache.js and Chrome DevTools
For those focusing on client-side implementations, integrating Mustache.js can simplify dynamic content rendering. When combined with Chrome DevTools, you can debug and fine-tune the user experience in real time.
Why Mustache.js?
Mustache.js is a simple templating engine that helps you insert calendar event data directly into HTML. This is super handy when you need to display meeting details or list attendees.
Simple Steps:
- Include Mustache.js in your project.
- Set up a template for meeting reminder messages.
- Fetch data from the Google Calendar API using JavaScript.
- Render the data with Mustache.js to create dynamic reminder messages that pop up when an attendee is marked as late.
Using Chrome DevTools for Debugging
Chrome DevTools makes it easier to inspect how your app interacts with the API. It allows you to:
- Monitor network requests to see if the API calls are successful.
- Check JavaScript console for any errors during the rendering process.
- Step through your code to see if the Mustache.js template is properly filled with data.
These tools are vital when developing in modern front-end environments, ensuring that your meeting reminder system functions smoothly even when handling real-time data.
Extra Resources and Tips
Beyond the immediate integration options, several additional tools and resources can broaden your integration horizons. Developers often refer to external resources and platforms to troubleshoot, learn, and update their projects.
Zapier and GitHub Resources
- Zapier is a great tool for automating tasks. It can help you create workflows that connect your calendar events with other apps, ensuring that if someone is late for a meeting, they receive an extra nudge.
- GitHub is an excellent resource for finding projects that have integrated the Google Calendar API with meeting reminder systems. Searching GitHub repositories can provide templates, code snippets, and best practices.
Using these platforms in tandem with your existing integrations will provide a more robust and fail-safe system.
Tips for Creating Meeting Reminders
Automatically pinging attendees who arrive late to meetings can dramatically improve meeting productivity. Here are some useful pointers:
- Automate Reminders:
When building with Google Calendar, always set up automated triggers that scan event timings. For instance, schedule a check five minutes into the meeting to identify late arrivals. - Customization:
Use custom add-ons to tailor the reminder message. Sometimes a gentle, friendly reminder works better than a strict notification. - Multimodal Alerts:
Consider using both email and in-app notifications. Adding multiple channels increases the chances of the attendee noticing the reminder. - Feedback Loop:
Allow recipients to respond to reminders. A simple “Got it” or “Running late” can be logged to further refine reminder triggers for future events.
Implementation Considerations
While it might seem overwhelming at first, breaking down the work into manageable parts helps a lot. You can start by implementing a basic calendar sync, and then gradually add features like meeting delay notifications and detailed error logging.
- Keep your code modular so that each piece (authentication, meeting sync, notification) can be tested and maintained separately.
- Document every step so that if you encounter a problem later, you have a trail of what worked and what didn’t.
- Utilize community forums for additional support. People on Stack Overflow have faced similar issues and often share practical workarounds.
For more detailed workflows specific to meeting reminders, checking out detailed guides like those on Calendar Reminder can offer templates and inspiration. Similarly, if you are interested in even more nuanced setups with notifications for calendar events, you might find useful pointers within projects such as Essential Staff Meeting Reminder Strategies.
Wrapping Up Practical Integration Steps
When setting up your Google Calendar API for automated meeting reminders, always start with a clear architecture:
- Begin with securing your API calls using OAuth2.
- Build error handlers that catch and log API issues.
- Integrate with your backend framework or no-code solution.
- Extend your functionality with custom add-ons via Apps Script.
- Ensure your front-end displays data clearly by using tools such as Mustache.js and Chrome DevTools.
Following these phases means your application will smoothly remind attendees if they are late to meetings. Whether you are using SpringBoot for a full-fledged web service or Bubble API Connector for rapid development, the key is to break everything down into simple, manageable steps.
Taking advantage of both community resources like Stack Overflow and automation platforms like Zapier gives you the flexibility to tailor your solution to exactly what your meeting environments need. With steady improvements and community feedback, even a somewhat disorganized approach can yield robust and flexible meeting reminder systems that ensure every meeting starts on time.
Conclusion
We started this guide by exploring how to handle the Google Calendar API setup and the OAuth2 process with clarity and ease. In short, learning to setup your API credentials is all about following clear instructions, taking your time with each step, and making use of helpful tools like the Google Developer Console and Chrome DevTools. One key insight is the importance of knowing where to find practical examples and community advice on platforms like Stack Overflow, which simplifies even the trickier parts of API integration. Another important takeaway is understanding that a bit of troubleshooting goes a long way, even if the process sometimes feels a bit messy or disorganized.
As you look forward and refine your meeting sync processes, remember that our expertise, especially in services like Meeting Reminders, can assist in automatically pinging attendees who arrive late to your meetings. Take these learnings, apply them in your projects, and never hesitate to seek help when needed, making each step a bit smoother and more effective.
Related Posts
Frequently Asked Questions (FAQs)
What is the Google Calendar API?
The Google Calendar API is a service that lets you access and control your Google Calendar. Its a simple way to create, view, and manage events right from your app or website. It works with OAuth2 for secure access, making it easier to integrate with your apps without sharing your passwords everywhere.
How do I set up OAuth2 for the Google Calendar API?
Setting up OAuth2 for the Google Calendar API means you need to register your project using the Google Developer Console and get your credentials. Once thats done, you configure your app to use these credentials to request access to calendar data. Its all about following the simple steps provided by Google Developers and testing with a small app before going full-scale.
What are some common issues with the Google Calendar API and how can I fix them?
When working with the Google Calendar API, you might run into permission problems or errors connecting to the API. A lot of times, the error stems from a misconfiguration in your OAuth2 setup or using outdated tokens. You can solve these issues by double-checking your credentials in the developer console and referring to communities like Stack Overflow for help.
How can I integrate the Google Calendar API with other tools?
The Google Calendar API works well with many other apps. For instance, you can connect it with a web app using frameworks like SpringBoot or even use it with automation tools like Zapier. By integrating it with other platforms and APIs, you can sync your events, update user calendars automatically, and even schedule meetings across multiple time zones.
How does Meeting Reminders automatically ping the attendees that arrive late to your meetings?
Our service uses the Google Calendar API to schedule events and then leverages custom scripts to detect attendees who are late. This automatic ping feature helps manage meeting delays by sending reminders or notifications when someone shows up late. Its a neat solution meant to streamline your meeting process without extra manual work.
Sources
- curl - Why is Google Calendar API (oauth2) responding with ‘Insufficient Permission’? - Stack Overflow
- Displaying a List of Events using Google Calendar API | by Piumal Rathnayake | Medium
- Google Calendar API use case - Plugins - Bubble Forum
- Google Calendar API don’t return shared calendars - Stack Overflow