· Ricardo Batista · 14 min read
Enhance Apps with Google Meets API Features
Learn how to enhance your apps with Google Meets API features to improve user experiences and simplify communication processes.
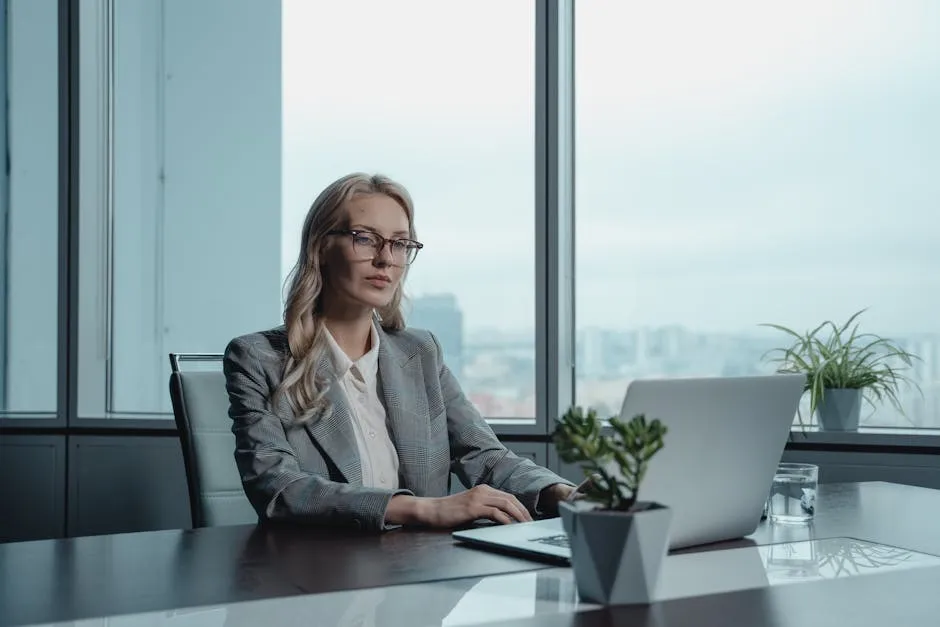
In the world of virtual interaction & teamwork, the Google Meets API shines as a strong instrument for combining video conferencing abilities into different programs. This piece explores the functions, advantages, & realistic uses of the Google Meets API, giving knowledge into how coders can utilize its characteristics to improve user encounters & simplify communication procedures.
Key Takeaways
- Blending the Google Meets API permits coders to effortlessly incorporate video conferencing capabilities into their apps.
- Understanding OAuth 2.0 authentication is crucial for accessing and utilizing the Google Meets API effectively.
Introduction to Google Meets API
What is Google Meets API?
The Google Meets API is a mighty instrument which permits coders to combine Google Meets video conferencing talents into their individual programs. This API furnishes a variety of functionalities, like generating & handling gatherings, obtaining meeting specifics, and integrating with additional Google Workspace implements such as Google Calendar & Gmail. Through utilizing the Google Meets API, coders can fashion seamless & efficient video conferencing encounters inside their programs, boosting user engagement & productivity.
To get started with the Google Meets API, you need to:
- Enable the API: Go to the Google Cloud Console, create a new project, and enable the Google Meets API.
- Establish Verification: Acquire OAuth 2.0 credentials to authenticate your program. This involves generating a client ID & client secret.
- Request API Data: Utilize the given endpoints to produce, handle, & get meeting info. You could utilize instruments like Postman to test your API requests.
For a detailed guide on how to set up and use the Google Meets API, you can refer to the official Google Developers documentation.
Benefits of Using Google Meets API
Integrating the Google Meets API into your application offers several benefits:
- Smooth Blending: The interface permits effortless blending with different Google Workspace instruments, like Google Calendar & Gmail. This guarantees that users can arrange & participate in gatherings straightforwardly from their current work processes.
- Improved User Interaction: Through incorporating video conferencing abilities directly into your program, you could offer a more unified & smooth user experience. Participants won’t have to switch between various platforms to attend meetings.
- Automation & Customization: The API allows automating meeting-related tasks, like sending reminders & managing meeting settings. This can save time & reduce manual effort. For instance, Meeting Reminders is a tool that notifies attendees when they aren’t showing up for meetings. By installing a Google add-on in your Google Calendar, Meeting Reminders automates the process of sending meeting reminders, ensuring your meetings start on time without needing manual follow-ups.
- Expandability: The Google Meets API is planned to deal with huge applications, making it appropriate for organizations of all dimensions. Whether you’re a little startup or a huge business, the API can uphold your video conferencing requirements.
For more information on how to effectively use the Google Meets API, you can check out our detailed guide on how to use Google Meets.
Getting Started with Google Meets API
Prerequisites and Requirements
Before getting into the combination of the Google Meets API, there are a couple of necessities & requirements you have to satisfy:
- Google Cloud Profile: You require a Google Cloud profile to get the Google Cloud Console & enable the Google Meets API. If you don’t possess one, you can register here.
- Payment Account: Make certain you’ve established a payment account in Google Cloud. Some API characteristics could necessitate billing to be activated.
- OAuth 2.0 Credentials: You’ll require OAuth 2.0 credentials to verify your app. This involves making a client ID & client secret.
- Basic Knowledge of REST APIs: Familiarity with REST APIs and HTTP requests will be beneficial.
Setting Up Your Google Cloud Project
Setting up your Google Cloud project is a crucial step in integrating the Google Meets API. Follow these steps to get started:
Create a New Project:
- Go to the Google Cloud Console.
- Click on the project dropdown at the top of the page and select “New Project”.
- Enter a project name and select your billing account. Click “Create”.
Enable the Google Meets API:
- In the Google Cloud Console, navigate to the “APIs & Services” section.
- Click on “Library” and search for “Google Meet API”.
- Click on the Google Meet API and then click “Enable”.
Set Up OAuth 2.0 Credentials:
- Go to the “Credentials” tab in the “APIs & Services” section.
- Click on “Create Credentials” and select “OAuth 2.0 Client ID”.
- Configure the consent screen by providing the necessary information.
- Create the OAuth 2.0 client ID by selecting the application type and providing the required details.
- Note down the client ID and client secret.
Install and Configure Meeting Reminders:
- Visit Meeting Reminders and install the Google add-on in your Google Calendar.
- Meeting Reminders will instantly notify participants when they aren’t appearing for gatherings, sparing you the trouble of manually messaging them each time they’re tardy.
By following these actions, you’ll get your Google Cloud venture arranged & prepared to incorporate the Google Meets API.
Blending the Google Meets API could seriously improve your app’s features, offering smooth video conferencing abilities. Furthermore, tools like Meeting Reminders can additionally simplify your meeting organization process, guaranteeing that your meetings begin promptly & run effortlessly.
Authentication and Authorization
OAuth 2.0 Overview
OAuth 2.0 is a standard way for apps to get restricted entry to user profiles on a web service. It works by letting the service that has the user profile verify the user & allowing third-party apps to access the user profile, without revealing the user’s login info.
In the situation of the Google Meets API, OAuth 2.0 is utilized to verify and permit your program to get Google Meet facilities on account of the client. This guarantees that your application can safely cooperate with Google Meet capabilities, like making and dealing with gatherings.
Implementing OAuth 2.0 for Google Meets API
Putting into action OAuth 2.0 for the Google Meets API necessitates numerous procedures. Here’s a detailed guide to assist you in commencing:
- Create OAuth 2.0 Credentials:
- Go to the Google Cloud Console.
- Navigate to the “APIs & Services” section and select “Credentials”.
- Click on “Create Credentials” and choose “OAuth 2.0 Client ID”.
- Set up the consent screen by giving the needed info, like the app name & approved domains.
- Make the OAuth 2.0 client ID by picking the application kind (like, Web application) & giving the needed info.
- Note down the client ID and client secret.
- Set Up OAuth 2.0 Flow:
Put into practice the OAuth 2.0 authorization process in your program. This usually involves redirecting the user to Google’s OAuth 2.0 server to grant permissions.
- Once the user grants permissions, Google will redirect them back to your application with an authorization code.
- Exchange the authorization code for an access token by making a POST request to Google’s token endpoint.
Here’s a sample code snippet in Python using the
requests
library:import requests def get_access_token(auth_code, client_id, client_secret, redirect_uri): token_url = "https://oauth2.googleapis.com/token" data = { 'code': auth_code, 'client_id': client_id, 'client_secret': client_secret, 'redirect_uri': redirect_uri, 'grant_type': 'authorization_code' } response = requests.post(token_url, data=data) return response.json()
- Use the Access Token:
Utilize the entry permit to create approved API demands to the Google Meets API. Incorporate the entry permit in the
Authorization
header of your HTTP demands.headers = { 'Authorization': f'Bearer {access_token}' } response = requests.get('https://www.googleapis.com/meet/v1/meetings', headers=headers)
Additionally, implements like Meeting Reminders can further boost your gathering administration cycle. Meeting Reminders pings participants when they aren’t appearing for gatherings, saving you the inconvenience of physically emailing them each time they’re late and. Simply set up the Google add-on in your Google Calendar & let Meeting Reminders do the remainder.
For more detailed instructions on setting up and using the Google Meets API, you can refer to our guide on how to use Google Meets.
Using Google Meets API
Creating and Configuring Meet Calls
Creating and configuring meet calls using the Google Meets API is straightforward. Here’s how you can do it:
- Create a Meeting:
Utilize the
make
endpoint to produce a fresh gathering. You must incorporate the required parameters like the gathering name, start period, & end period.- Example request in Python:
import requests def create_meeting(access_token, title, start_time, end_time): url = "https://www.googleapis.com/calendar/v3/calendars/primary/events" headers = { 'Authorization': f'Bearer {access_token}', 'Content-Type': 'application/json' } data = { "summary": title, "start": {"dateTime": start_time}, "end": {"dateTime": end_time}, "conferenceData": { "createRequest": { "requestId": "sample123", "conferenceSolutionKey": {"type": "hangoutsMeet"} } } } response = requests.post(url, headers=headers, json=data) return response.json()
- Configure Meeting Settings:
- Ya can set up different stuff like who can join, if it’s recorded, & other things by changing the meeting thing.
Retrieving Meeting Information
To obtain data regarding a particular gathering, you are able to utilize the get
endpoint. This permits you to retrieve specifics like the meeting ID, attendees, & meeting URL.
- Example request in Python:
def get_meeting_info(access_token, meeting_id):
url = f"https://www.googleapis.com/calendar/v3/calendars/primary/events/{meeting_id}"
headers = {
'Authorization': f'Bearer {access_token}'
}
response = requests.get(url, headers=headers)
return response.json()
Accessing Meeting Artifacts
Meeting artifacts like recordings, chat logs, & attendance reports can be gotten through the API. This is especially handy for post-meeting analysis and record-keeping.
- Example request to retrieve meeting recordings:
def get_meeting_recordings(access_token, meeting_id):
url = f"https://www.googleapis.com/drive/v3/files?q='{meeting_id}' in parents"
headers = {
'Authorization': f'Bearer {access_token}'
}
response = requests.get(url, headers=headers)
return response.json()
Subscribing to Real-Time Updates
Joining to live updates permits your program to get notifications regarding modifications to gatherings, like fresh participants connecting or meeting cancellations. This could be accomplished utilizing webhooks.
Set Up a Webhook:
- Register a webhook URL with the Google Meets API to receive real-time updates.
- Example request in Python:
def subscribe_to_updates(access_token, webhook_url): url = "https://www.googleapis.com/calendar/v3/calendars/primary/events/watch" headers = { 'Authorization': f'Bearer {access_token}', 'Content-Type': 'application/json' } data = { "id": "unique-channel-id", "type": "web_hook", "address": webhook_url } response = requests.post(url, headers=headers, json=data) return response.json()
By utilizing these capabilities, you can develop a sturdy & interactive video conferencing encounter inside your program.
Additionally, implements like Meeting Reminders can further boost your gathering supervision procedure. Meeting Reminders pings participants when they aren’t appearing for gatherings, saving you the inconvenience of physically messaging them each time they’re tardy. Simply set up the Google add-on in your Google Calendar & let Meeting Reminders handle the remainder.
Integrating Google Meets API with Other Tools
Integration with CRM Systems
Blending the Google Meets Application Programming Interface (API) alongside Customer Relationship Administration (CRM) frameworks could massively upgrade your client collaborations by permitting consistent video conferencing straightforwardly from your CRM stage. Here’s the way you can accomplish this:
Pick a CRM Platform: Well-known CRM platforms like Salesforce, HubSpot, & Zoho CRM provide APIs that could be combined with the Google Meets API.
Create a Meeting from CRM:
- Use the Google Meets API to create a meeting when a new lead or contact is added to your CRM.
- Example in Python:
import requests def create_meeting_from_crm(access_token, contact_email, title, start_time, end_time): url = "https://www.googleapis.com/calendar/v3/calendars/primary/events" headers = { 'Authorization': f'Bearer {access_token}', 'Content-Type': 'application/json' } data = { "summary": title, "start": {"dateTime": start_time}, "end": {"dateTime": end_time}, "attendees": [{"email": contact_email}], "conferenceData": { "createRequest": { "requestId": "crm-meeting-123", "conferenceSolutionKey": {"type": "hangoutsMeet"} } } } response = requests.post(url, headers=headers, json=data) return response.json()
Automate Meeting Reminders:
- Utilize Meeting Reminders to mechanically notify participants when they aren’t appearing for gatherings. This could save you time & guarantee your meetings commence promptly. Simply set up the Google extension in your Google Calendar & allow Meeting Reminders to handle the remaining tasks.
Integration with Project Management Tools
Blending the Google Meets API alongside task management platforms like Asana, Trello, & Jira can simplify your project processes by allowing video conferencing directly inside your project management setting. Here’s how to accomplish it:
Choose a Project Management Tool: Select a project management tool that supports API integration.
Create a Meeting from Project Tasks:
- Use the Google Meets API to create a meeting when a new task or project is created.
- Example in Python:
import requests def create_meeting_from_task(access_token, task_name, start_time, end_time): url = "https://www.googleapis.com/calendar/v3/calendars/primary/events" headers = { 'Authorization': f'Bearer {access_token}', 'Content-Type': 'application/json' } data = { "summary": task_name, "start": {"dateTime": start_time}, "end": {"dateTime": end_time}, "conferenceData": { "createRequest": { "requestId": "task-meeting-123", "conferenceSolutionKey": {"type": "hangoutsMeet"} } } } response = requests.post(url, headers=headers, json=data) return response.json()
Sync Meeting Details with Tasks:
Make sure the meeting info like the link & time is automatically put in the task details or comments.
- Example in Python:
def update_task_with_meeting_link(task_id, meeting_link): # Assuming you have a function to update tasks in your project management tool update_task(task_id, {"description": f"Meeting Link: {meeting_link}"})
Combining the Google Meets API alongside your CRM & project management tools, you could establish a more streamlined and unified workflow, improving both client interactions and project management.
Additionally, implements like Meeting Reminders can additionally simplify your gathering administration cycle. Meeting Reminders pings participants when they aren’t appearing for gatherings, saving you the inconvenience of physically messaging them each time they’re tardy. Simply introduce the Google add-on in your Google Calendar & let Meeting Reminders do the remainder.
Troubleshooting and Best Practices
Common Issues and Solutions
When working with the Google Meets API, you may encounter some common issues. Here are a few and their solutions:
- Authentication Errors:
- Issue: Receiving a 401 Unauthorized error.
Solution: Make certain your OAuth 2.0 credentials are accurate & the access token is legitimate. You can renew the token if it has run out.
def refresh_access_token(client_id, client_secret, refresh_token): url = "https://oauth2.googleapis.com/token" data = { 'client_id': client_id, 'client_secret': client_secret, 'refresh_token': refresh_token, 'grant_type': 'refresh_token' } response = requests.post(url, data=data) return response.json()
- Quota Limits:
- Issue: Hitting API quota limits.
- Solution: Keep track of your API usage in the Google Cloud Console & ask for a higher quota if needed. Enhance your API calls to cut down on unnecessary requests.
- Meeting Creation Failures:
- Issue: Unable to create a meeting.
Solution: Make certain that every necessary field is included within the API request & check for any validation mistakes in the response.
def create_meeting(access_token, title, start_time, end_time): url = "https://www.googleapis.com/calendar/v3/calendars/primary/events" headers = { 'Authorization': f'Bearer {access_token}', 'Content-Type': 'application/json' } data = { "summary": title, "start": {"dateTime": start_time}, "end": {"dateTime": end_time}, "conferenceData": { "createRequest": { "requestId": "sample123", "conferenceSolutionKey": {"type": "hangoutsMeet"} } } } response = requests.post(url, headers=headers, json=data) return response.json()
Best Practices for Using Google Meets API
To ensure a smooth and efficient integration with the Google Meets API, follow these best practices:
- Use OAuth 2.0 Securely:
- Constantly utilize OAuth 2.0 for verification & approval. Safely keep your client ID, client secret, & tokens. Dodge hardcoding them in your app.
- Handle Errors Gracefully:
- Put in place strong mistake handling in your app. Look for common HTTP status codes & give users clear error messages.
- Optimize API Calls:
- Decrease the quantity of API requests by storing responses where feasible. Utilize batch demands to lessen the number of HTTP requests.
- Monitor API Usage:
- Frequently check your API utilization in the Google Cloud Dashboard. Establish notifications for quota boundaries & other crucial measurements.
- Automate Meeting Reminders:
- Utilize instruments like Meeting Reminders to mechanize the procedure of dispatching notifications to participants. Meeting Reminders pings participants when they aren’t appearing for gatherings, sparing you the inconvenience of physically messaging them each time they’re tardy. Simply set up the Google add-on in your Google Calendar & let Meeting Reminders handle the remainder.
By adhering to these optimal techniques, you can guarantee a trustworthy & streamlined integration with the Google Meets API. For more thorough directions on utilizing the Google Meets API, you could consult our guide on how to employ Google Meets.
Conclusion
To summarize, combining Google Meets API within your programs could transform how you handle video conferencing & teamwork. Following the instructions in this guide, you can effortlessly blend Google Meets robust capabilities into your processes, boosting communication & output. From authentication & approval to generating & setting up meet calls, utilizing Google Meets API unlocks a universe of opportunities for developers & businesses. Adhering to recommended practices & resolving typical problems, you can guarantee a smooth integration & harness video conferencing tech’s full potential. Embrace Google Meets API power to elevate your virtual communication.
Frequently Asked Questions (FAQs)
What is the Google Meets API?
How can I get started with the Google Meets API?
To begin utilizing the Google Meets API, you require possessing a Google Cloud account, establishing OAuth 2.0 for verification, & acquainting yourself with the API documentation.
What are the benefits of integrating the Google Meets API?
Blending the Google Meets API allows smooth video conferencing inside your programs, improving teamwork & interaction between people.
Can I integrate Google Meets API with CRM systems?
Yup, ya can combine the Google Meets API with Customer Connection Administration (CRM) frameworks to make video conferencing straightforwardly from your CRM stage.
What are some common issues when using the Google Meets API?
Common problems when utilizing the Google Meets API comprise authentication mistakes, meeting formation breakdowns, & authorization-linked troubles. Resolving these can assist guarantee seamless incorporation & utilization.